Avatar
A graphical representation of a user or entity, often an image or initials displayed in a circular container.
Sizes
Avatars come in five sizes.
Example: Color avatar
import { Avatar, Size } from '@skiff-org/skiff-ui';
<Avatar label='Alice' size={Size.LARGE} />
<Avatar label='Alice' size={Size.X_MEDIUM} />
<Avatar label='Alice' size={Size.MEDIUM} />
<Avatar label='Alice' size={Size.SMALL} />
<Avatar label='Alice' size={Size.X_SMALL} />
Colors
Color is based on the full string passed to the Avatar
but can be overwritten with the color
prop.
Example: Color avatar
import { Avatar, Size } from '@skiff-org/skiff-ui';
<Avatar color='pink' label='Alice' size={Size.LARGE} />
<Avatar color='yellow' label='Alice' size={Size.LARGE} />
<Avatar color='red' label='Alice' size={Size.LARGE} />
<Avatar color='orange' label='Alice' size={Size.LARGE} />
<Avatar color='green' label='Alice' size={Size.LARGE} />
<Avatar color='blue' label='Alice' size={Size.LARGE} />
<Avatar color='dark-blue' label='Alice' size={Size.LARGE} />
Initial Avatar
Avatars will display just the first character when given a label
.
Example: Initial avatar
import { Avatar, Size } from '@skiff-org/skiff-ui';
<Avatar label='Alice' size={Size.LARGE} />
<Avatar label='Alice' size={Size.X_MEDIUM} />
<Avatar label='Alice' />
<Avatar label='Alice' size={Size.SMALL} />
<Avatar label='Alice' size={Size.X_SMALL} />
Icon Avatar
You can display any Icon
from the Skiff UI Icon library inside Avatars.
Example: Icon avatar
import { Avatar, Icon, Size } from '@skiff-org/skiff-ui';
<Avatar icon={Icon.Lock} size={Size.LARGE} />
<Avatar icon={Icon.Lock} size={Size.X_MEDIUM} />
<Avatar icon={Icon.Lock} />
<Avatar icon={Icon.Lock} size={Size.SMALL} />
<Avatar icon={Icon.Lock} size={Size.X_SMALL} />
Photo Avatar
Images can also be passed into the Avatar
component.
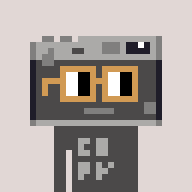
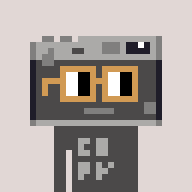
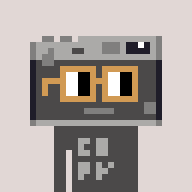
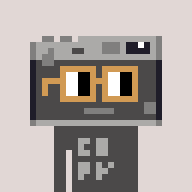
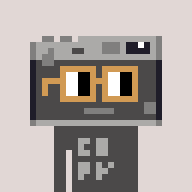
Example: Photo avatar
import { Avatar, Size } from '@skiff-org/skiff-ui';
<Avatar imgSrc={ProfilePic} size={Size.LARGE} />
<Avatar imgSrc={ProfilePic} size={Size.X_MEDIUM} />
<Avatar imgSrc={ProfilePic} />
<Avatar imgSrc={ProfilePic} size={Size.SMALL} />
<Avatar imgSrc={ProfilePic} size={Size.X_SMALL} />
Active states
Show badges when user is active or online. Fade Avatar
when a user is inactive or offline.
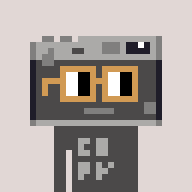
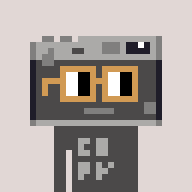
Example: Active states
import { Avatar, Size} from '@skiff-ui';
<Avatar showBadge imgSrc={ProfilePic} size={Size.LARGE} />
<Avatar active={false} imgSrc={ProfilePic} size={Size.LARGE} />
<Avatar disabled size={Size.LARGE} />
Rounded avatar
Display the Avatar
with a circlular border radius.
Example: Photo avatar
import { Avatar, Size } from '@skiff-org/skiff-ui';
<Avatar rounded label='Alice' size={Size.LARGE} />
Properties
- Name
active
- Type
- boolean
- Description
Set to false when the user is offline
- Name
background
- Type
- string
- Description
Set the background color without updating the text color.
- Name
badgeColor
- Type
- AccentColor
- Description
Set the badge color.
- Name
badgeIcon
- Type
- Icon
- Description
Show an icon inside the badge.
- Name
badgeSize
- Type
- number
- Description
Override the default badge size.
- Name
color
- Type
- AccentColor
- Description
Set the color of the avatar. Controls background and text color together.
- Name
disabled
- Type
- boolean
- Description
Set the avatar to a disabled (grayed out) state.
- Name
forceTheme
- Type
- ThemeMode
- Description
Force the theme (dark or light mode) of the avatar, regardless of app theme.
- Name
icon
- Type
- Icon
- Description
Set the icon inside the Avatar.
- Name
imgSrc
- Type
- string
- Description
Source data for setting the avatar image.
- Name
label
- Type
- string
- Description
Label value of the avatar. Only the first character is shown.
- Name
rounded
- Type
- boolean
- Description
Display a circular avatar instead of square.
- Name
size
- Type
- Size
- Description
The size for the avatar.
- Name
onClick
- Type
- (e: React.MouseEvent) => void
- Description
Trigger an action when clicking the avatar.