Dropdown
Presents a menu-like list of options when activated, providing a compact way for users to choose or perform actions.
Overview
Attach a Button to a Dropdown via the buttonRef and portal props. Add DropdownItem children to add options to the Dropdown menu.
Example: Dropdown
import { Button, Dropdown, DropdownItem } from '@skiff-org/skiff-ui';
const buttonRef = useRef(null);
const [showDropdown, setShowDropdown] = useState(false);
<>
<Button
ref={buttonRef}
forceTheme={theme}
type={Type.SECONDARY}
onClick={()=>{setShowDropdown(!showDropdown) }}
>
Click to open
</Button>
<Dropdown
portal
gapFromAnchor={8}
buttonRef={buttonRef}
setShowDropdown={setShowDropdown}
showDropdown={showDropdown}
>
<DropdownItem
icon={Icon.Map}
label='Change plan'
onClick={()=>{}}
value='change-plan'
/>
<DropdownItem
color='destructive'
icon={Icon.Trash}
label='Delete'
onClick={()=>{}}
value='delete-plan'
/>
</Dropdown>
</>
Dropdown without Portal
For the main action the user should take. Generative or forward progressing.
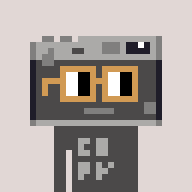
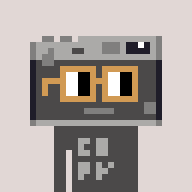
Example: Dropdown without Portal
import { Avatar, Dropdown, DropdownItem, ThemeMode } from '@skiff-org/skiff-ui';
<Dropdown showDropdown={true}>
<DropdownItem
icon={<Avatar imgSrc={ProfilePic} />}
onClick={()=>{}}
value='alice'
/>
<Divider forceTheme={ThemeMode.DARK} />
<DropdownItem
icon={<Avatar imgSrc={ProfilePic} />}
onClick={()=>{}}
value=bob'
/>
</Dropdown>
Properties
- Name
buttonRef
- Type
- React.MutableRefObject<HTMLDivElement | null>
- Description
Reference to the
Button
that triggers theDropdown
to open.
- Name
children
- Type
- React.ReactNode | Array<DropdownItem>
- Description
Children that are passed into the Dropdown as items.
- Name
className
- Type
- string
- Description
Override class styles.
- Name
customAnchor
- Type
- { x: number; y: number }
- Description
Set the
Dropdown
anchor to a specified position (e.g.MouseEvent
position).
- Name
fullWidth
- Type
- boolean
- Description
Whether the
Dropdown
should take the full width of theDropdown
anchor.
- Name
gapFromAnchor
- Type
- number
- Description
Created a gap of the specified of px between the anchor and the dropdown.
- Name
highlightedIdx
- Type
- number
- Description
Force the selection state of the item at the
highlightedIdx
in the dropdown.
- Name
isSubmenu
- Type
- boolean
- Description
Whether the
Dropdown
is a sub-menu within anotherDropdown
.
- Name
maxHeight
- Type
- number
- Description
Max
Dropdown
height for overflow.
- Name
maxWidth
- Type
- number
- Description
Maximum
Dropdown
width.
- Name
minWidth
- Type
- number
- Description
Minimum
Dropdown
width.
- Name
portal
- Type
- boolean
- Description
Portal the
Dropdown
to the root of theDOM
.
- Name
setHighlightedIdx
- Type
- (idx?: number) => void
- Description
Update the
highlightedIdx
value for forcing a selection state.
- Name
setShowDropdown
- Type
- (open: boolean) => void
- Description
Control whether the
Dropdown
is open or closed.
- Name
showDropdown
- Type
- boolean
- Description
Whether the
Dropdown
is open or closed.
- Name
width
- Type
- number
- Description
Custom
Dropdown
width.
- Name
zIndex
- Type
- number
- Description
Custom
Dropdown
z-itemIndex.
DropdownItem Properties
- Name
label
- Type
- string
- Description
Text to display for dropdown item.
- Name
active
- Type
- boolean
- Description
Controls the DropdownItem's active state (i.e. displays check).
- Name
color
- Type
- DropdownItemColor
- Description
DropdownItem label color.
- Name
customLabel
- Type
- JSX.Element
- Description
DropdownItem label color.
- Name
disabled
- Type
- boolean
- Description
Controls the DropdownItem's disabled state (i.e. non-interactive).
- Name
endElement
- Type
- JSX.Element
- Description
Component displayed at the end of the dropdown item row.
- Name
highlight
- Type
- boolean
- Description
Controls the DropdownItem's hover state (force background color). Used for keyboard navigation.
- Name
icon
- Type
- Icon
- Description
Icon displayed at start of DropdownItem.
- Name
iconColor
- Type
- IconColor
- Description
Start icon color.
- Name
scrollIntoView
- Type
- boolean
- Description
Whether the dropdown item should be scrolled into view. Used for keyboard navigation.
- Name
size
- Type
- DropdownItemSize
- Description
Size of the DropdownItem component.
- Name
startElement
- Type
- JSX.Element
- Description
Element displayed at the start of the DropdownItem.
- Name
value
- Type
- string
- Description
Value for controlling active state dropdown in menu.
- Name
onClick
- Type
- (e: React.MouseEvent<HTMLDivElement, MouseEvent>) => void | Promise<void>
- Description
Trigger an action when the DropdownItem is clicked.
- Name
onHover
- Type
- () => void
- Description
Trigger an action when the DropdownItem is hovered.
- Name
isLast
- Type
- boolean
- Description
Hide the bottom border if DropdownItem is last in Dropdown.