Chip
A compact component displaying small information or attributes, commonly used for tags, categories, or short descriptions.
Default state
A compact component displaying small information or attributes, commonly used for tags, categories, or short descriptions.
Example: Default Chip
import { Chip, Icon, Size } from '@skiff-org/skiff-ui';
<Chip label='Reply' icon={Icon.Reply} size={Size.LARGE} />
<Chip label='Reply' icon={Icon.Reply} />
<Chip label='Reply' icon={Icon.Reply} size={Size.SMALL} />
Avatar chip
Add avatars for displaying user information in a chip.
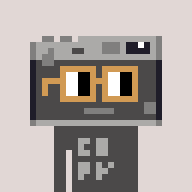
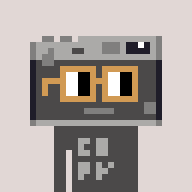
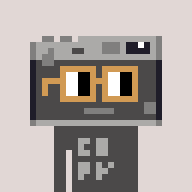
Example: Default Chip
import { Avatar, Chip, Size } from '@skiff-org/skiff-ui';
<Chip label='Alice' icon={<Avatar imgSrc={ProfilePic} />} size={Size.LARGE} />
<Chip label='Alice' icon={<Avatar imgSrc={ProfilePic} />} />
<Chip label='Alice' icon={<Avatar imgSrc={ProfilePic} />} size={Size.SMALL} />
Tag chip
Adding an onDelete
will render a tag
chip. Tags have a clickable close button and hover states.
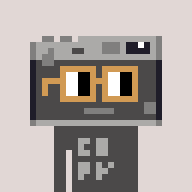
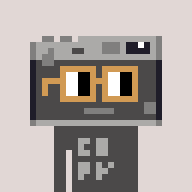
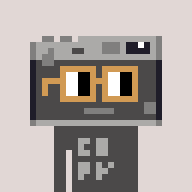
Example: Default Chip
import { Avatar, Chip, Size } from '@skiff-org/skiff-ui';
<Chip label='Alice' icon={<Avatar imgSrc={ProfilePic} />} onDelete={()=>{}} size={Size.LARGE} />
<Chip label='Alice' icon={<Avatar imgSrc={ProfilePic} />} onDelete={()=>{}} />
<Chip label='Alice' icon={<Avatar imgSrc={ProfilePic} />} onDelete={()=>{}} size={Size.SMALL} />
Properties
- Name
color
- Type
- Color
- Description
Override the text color for the chip.
- Name
containerColor
- Type
- AccentColor
- Description
Override the container color for the chip.
- Name
destructive
- Type
- boolean
- Description
Show a destructive (i.e. red) variant of the chip.
- Name
label
- Type
- string
- Description
Label displayed inside the chip.
- Name
icon
- Type
- Icon | Avatar
- Description
Icon or Avatar displayed at the start of the chip.
- Name
endIcon
- Type
- Icon
- Description
Icon at the end of the chip (only applicable for tag type).
- Name
size
- Type
- Size
- Description
The size for the avatar.
- Name
noBorder
- Type
- boolean
- Description
Hide the chip border.
- Name
onClick
- Type
- (e: React.MouseEvent) => void
- Description
Trigger an action when part of the chip container is clicked.
- Name
onDelete
- Type
- (e: React.MouseEvent) => void
- Description
Triggered when the x icon on an input tag is clicked. When defined renders Chip as "tag" type.
- Name
tooltip
- Type
- string
- Description
Display a tooltip label on hover.
- Name
typographyWeight
- Type
- TypographyWeight
- Description
Set the font weight of the label inside the chip.